Starting place Tale
It began a couple of years in the past when participants of one in every of my groups requested,
“what development must we undertake for dependency injection (DI)”?
The workforce’s stack was once Typescript on Node.js, no longer one I used to be extraordinarily acquainted with, so I
inspired them to paintings it out for themselves. I used to be disenchanted to be informed
a while later that workforce had determined, in impact, to not make a decision, leaving
at the back of a plethora of patterns for wiring modules in combination. Some builders
used manufacturing unit strategies, others guide dependency injection in root modules,
and a few items at school constructors.
The consequences had been lower than very best: a hodgepodge of object-oriented and
purposeful patterns assembled in several techniques, each and every requiring an excessively
other way to trying out. Some modules had been unit testable, others
lacked access issues for trying out, so easy good judgment required complicated HTTP-aware
scaffolding to workout fundamental capability. Maximum significantly, adjustments in
one a part of the codebase now and again brought about damaged contracts in unrelated spaces.
Some modules had been interdependent throughout namespaces; others had utterly flat collections of modules with
no difference between subdomains.
With the good thing about hindsight, I persevered to suppose
about that unique resolution: what DI development must now we have picked.
In the long run I got here to a conclusion: that was once the incorrect query.
Dependency injection is a way, no longer an finish
On reflection, I must have guided the workforce against asking a distinct
query: what are the specified qualities of our codebase, and what
approaches must we use to reach them? I want I had advocated for the
following:
- discrete modules with minimum incidental coupling, even at the price of some reproduction
varieties - industry good judgment this is saved from intermingling with code that manages the delivery,
like HTTP handlers or GraphQL resolvers - industry good judgment exams that aren’t transport-aware or have complicated
scaffolding - exams that don’t wreck when new fields are added to varieties
- only a few varieties uncovered outdoor in their modules, or even fewer varieties uncovered
outdoor of the directories they inhabit.
Over the previous couple of years, I have settled on an method that leads a
developer who adopts it towards those qualities. Having come from a
Test-Driven Development (TDD) background, I naturally get started there.
TDD encourages incrementalism however I sought after to head even additional,
so I’ve taken a minimalist “function-first” way to module composition.
Reasonably than proceeding to explain the method, I can display it.
What follows is an instance internet carrier constructed on a quite easy
structure in which a controller module calls area good judgment which in flip
calls repository purposes within the patience layer.
The issue description
Believe a person tale that appears one thing like this:
As a registered person of RateMyMeal and a would-be eating place patron who
does not know what is to be had, I want to be supplied with a ranked
set of really useful eating places in my area in response to different patron rankings.
Acceptance Standards
- The eating place checklist is ranked from probably the most to the least
really useful. - The score procedure contains the next attainable score
ranges: - superb (2)
- above moderate (1)
- moderate (0)
- underneath moderate (-1)
- horrible (-2).
- The total score is the sum of all particular person rankings.
- Customers regarded as “depended on” get a 4X multiplier on their
score. - The person should specify a town to restrict the scope of the returned
eating place.
Construction an answer
I’ve been tasked with development a REST carrier the use of Typescript,
Node.js, and PostgreSQL. I get started through development an excessively coarse integration
as a walking skeleton that defines the
limitations of the issue I want to remedy. This check makes use of as a lot of
the underlying infrastructure as imaginable. If I exploit any stubs, it is
for third-party cloud suppliers or different products and services that can not be run
in the neighborhood. Even then, I exploit server stubs, so I will be able to use actual SDKs or
community shoppers. This turns into my acceptance check for the duty to hand,
preserving me centered. I can simplest quilt one “satisfied trail” that workouts the
fundamental capability because the check might be time-consuming to construct
robustly. I’m going to in finding more cost effective techniques to check edge instances. For the sake of
the item, I suppose that I’ve a skeletal database construction that I will be able to
adjust if required.
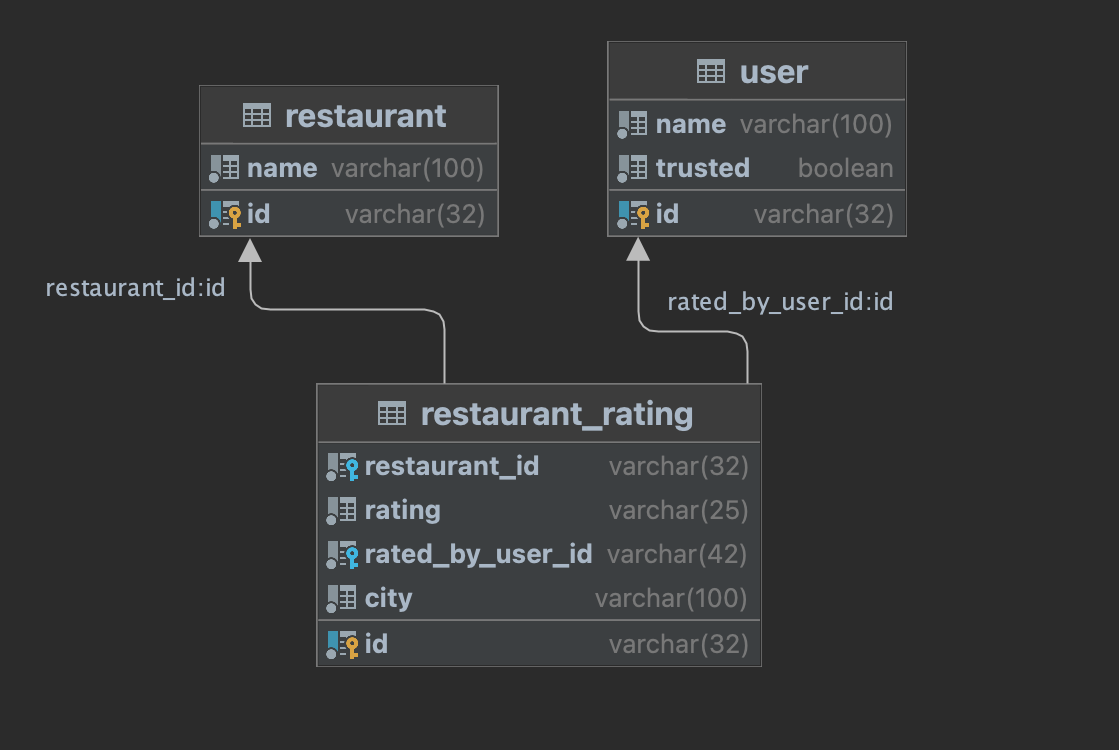
Exams typically have a given/when/then
construction: a suite of
given situations, a taking part motion, and a verified outcome. I like to
get started at when/then
and again into the given
to lend a hand me center of attention the issue I am looking to remedy.
“When I name my advice endpoint, then I be expecting to get an OK reaction
and a payload with the top-rated eating places in response to our rankings
set of rules”. In code which may be:
check/e2e.integration.spec.ts…
describe("the eating places endpoint", () => { it("ranks through the advice heuristic", async () => { const reaction = anticipate axios.get<ResponsePayload>( ➀ "http://localhost:3000/vancouverbc/eating places/really useful", { timeout: 1000 }, ); be expecting(reaction.standing).toEqual(200); const information = reaction.information; const returnRestaurants = information.eating places.map(r => r.identity); be expecting(returnRestaurants).toEqual(["cafegloucesterid", "burgerkingid"]); ➁ }); }); variety ResponsePayload = { eating places: { identity: string; title: string }[]; };
There are a few main points value calling out:
Axios
is the HTTP shopper library I have selected to make use of.
The Axiosget
operate takes a kind argument
(ResponsePayload
) that defines the predicted construction of
the reaction information. The compiler will ensure that all makes use of of
reaction.information
agree to that variety, then again, this test can
simplest happen at compile-time, so can not ensure the HTTP reaction frame
in reality accommodates that construction. My assertions will wish to do
that.- Reasonably than checking all the contents of the returned eating places,
I simplest test their ids. This small element is planned. If I test the
contents of all the object, my check turns into fragile, breaking if I
upload a brand new box. I need to write a check that may accommodate the herbal
evolution of my code whilst on the identical time verifying the particular situation
I am occupied with: the order of the eating place record.
With out my given
situations, this check is not very precious, so I upload them subsequent.
check/e2e.integration.spec.ts…
describe("the eating places endpoint", () => { let app: Server | undefined; let database: Database | undefined; const customers = [ { id: "u1", name: "User1", trusted: true }, { id: "u2", name: "User2", trusted: false }, { id: "u3", name: "User3", trusted: false }, ]; const eating places = [ { id: "cafegloucesterid", name: "Cafe Gloucester" }, { id: "burgerkingid", name: "Burger King" }, ]; const ratingsByUser = [ ["rating1", users[0], eating places[0], "EXCELLENT"], ["rating2", users[1], eating places[0], "TERRIBLE"], ["rating3", users[2], eating places[0], "AVERAGE"], ["rating4", users[2], eating places[1], "ABOVE_AVERAGE"], ]; beforeEach(async () => { database = anticipate DB.get started(); const shopper = database.getClient(); anticipate shopper.attach(); take a look at { // GIVEN // Those purposes do not exist but, however I'm going to upload them in a while for (const person of customers) { anticipate createUser(person, shopper); } for (const eating place of eating places) { anticipate createRestaurant(eating place, shopper); } for (const score of ratingsByUser) { anticipate createRatingByUserForRestaurant(score, shopper); } } in spite of everything { anticipate shopper.finish(); } app = anticipate server.get started(() => Promise.unravel({ serverPort: 3000, ratingsDB: { ...DB.connectionConfiguration, port: database?.getPort(), }, }), ); }); afterEach(async () => { anticipate server.forestall(); anticipate database?.forestall(); }); it("ranks through the advice heuristic", async () => { // .. snip
My given
situations are carried out within the beforeEach
operate.
incorporates the addition of extra exams must
beforeEach
I want to make the most of the similar setup scaffold and helps to keep the pre-conditions
cleanly impartial of the remainder of the check. You’ll be able to understand numerous
anticipate
calls. Years of revel in with reactive platforms
like Node.js have taught me to outline asynchronous contracts for all
however probably the most straight-forward purposes.
The rest that finally ends up IO-bound, like a database name or record learn,
must be asynchronous and synchronous implementations are really easy to
wrap in a Promise, if essential. Against this, opting for a synchronous
contract, then discovering it must be async is a far uglier downside to
remedy, as we will see later.
I have deliberately deferred developing specific varieties for the customers and
eating places, acknowledging I do not know what they seem like but.
With Typescript’s structural typing, I will be able to proceed to defer developing that
definition and nonetheless get the good thing about type-safety as my module APIs
start to solidify. As we will see later, this can be a important method during which
modules may also be saved decoupled.
At this level, I’ve a shell of a check with check dependencies
lacking. The following degree is to flesh out the ones dependencies through first development
stub purposes to get the check to collect after which enforcing those helper
purposes. That may be a non-trivial quantity of labor, however it is also extremely
contextual and out of the scope of this newsletter. Suffice it to mention that it
will typically include:
- beginning up dependent products and services, comparable to databases. I typically use testcontainers to run dockerized products and services, however those may
even be community fakes or in-memory parts, no matter you favor. - fill within the
create...
purposes to pre-construct the entities required for
the check. In terms of this situation, those are SQLINSERT
s. - get started up the carrier itself, at this level a easy stub. We will dig a
little extra into the carrier initialization since it is germaine to the
dialogue of composition.
If you have an interest in how the check dependencies are initialized, you’ll be able to
see the results within the GitHub repo.
Sooner than transferring on, I run the check to verify it fails as I’d
be expecting. As a result of I’ve no longer but carried out my carrier
get started
, I be expecting to obtain a connection refused error when
making my http request. With that showed, I disable my giant integration
check, since it is not going to move for some time, and dedicate.
Directly to the controller
I typically construct from the outdoor in, so my subsequent step is to
deal with the principle HTTP dealing with operate. First, I’m going to construct a controller
unit check. I get started with one thing that guarantees an empty 200
reaction with anticipated headers:
check/restaurantRatings/controller.spec.ts…
describe("the rankings controller", () => {
it("supplies a JSON reaction with rankings", async () => {
const ratingsHandler: Handler = controller.createTopRatedHandler();
const request = stubRequest();
const reaction = stubResponse();
anticipate ratingsHandler(request, reaction, () => {});
be expecting(reaction.statusCode).toEqual(200);
be expecting(reaction.getHeader("content-type")).toEqual("utility/json");
be expecting(reaction.getSentBody()).toEqual({});
});
});
I have already began to do some design paintings that may lead to
the extremely decoupled modules I promised. Many of the code is reasonably
standard check scaffolding, however in case you glance intently on the highlighted operate
name it will strike you as peculiar.
This small element is step one towards
partial application,
or purposes returning purposes with context. Within the coming paragraphs,
I’m going to display the way it turns into the root upon which the compositional method is constructed.
Subsequent, I construct out the stub of the unit below check, this time the controller, and
run it to make sure my check is working as anticipated:
src/restaurantRatings/controller.ts…
export const createTopRatedHandler = () => { go back async (request: Request, reaction: Reaction) => {}; };
My check expects a 200, however I am getting no calls to standing
, so the
check fails. A minor tweak to my stub it is passing:
src/restaurantRatings/controller.ts…
export const createTopRatedHandler = () => { go back async (request: Request, reaction: Reaction) => { reaction.standing(200).contentType("utility/json").ship({}); }; };
I dedicate and transfer directly to fleshing out the check for the predicted payload. I
do not but know precisely how I can care for the information get entry to or
algorithmic a part of this utility, however I do know that I want to
delegate, leaving this module to not anything however translate between the HTTP protocol
and the area. I additionally know what I need from the delegate. Particularly, I
need it to load the top-rated eating places, no matter they’re and anyplace
they arrive from, so I create a “dependencies” stub that has a operate to
go back the end eating places. This turns into a parameter in my manufacturing unit operate.
check/restaurantRatings/controller.spec.ts…
variety Eating place = { identity: string }; variety RestaurantResponseBody = { eating places: Eating place[] }; const vancouverRestaurants = [ { id: "cafegloucesterid", name: "Cafe Gloucester", }, { id: "baravignonid", name: "Bar Avignon", }, ]; const topRestaurants = [ { city: "vancouverbc", restaurants: vancouverRestaurants, }, ]; const dependenciesStub = { getTopRestaurants: (town: string) => { const eating places = topRestaurants .clear out(eating places => { go back eating places.town == town; }) .flatMap(r => r.eating places); go back Promise.unravel(eating places); }, }; const ratingsHandler: Handler = controller.createTopRatedHandler(dependenciesStub); const request = stubRequest().withParams({ town: "vancouverbc" }); const reaction = stubResponse(); anticipate ratingsHandler(request, reaction, () => {}); be expecting(reaction.statusCode).toEqual(200); be expecting(reaction.getHeader("content-type")).toEqual("utility/json"); const despatched = reaction.getSentBody() as RestaurantResponseBody; be expecting(despatched.eating places).toEqual([ vancouverRestaurants[0], vancouverRestaurants[1], ]);
With so little data on how the getTopRestaurants
operate is carried out,
how do I stub it? I do know sufficient to design a fundamental shopper view of the contract I have
created implicitly in my dependencies stub: a easy unbound operate that
asynchronously returns a suite of Eating places. This contract could be
fulfilled through a easy static operate, one way on an object example, or
a stub, as within the check above. This module does not know, does not
care, and does not must. It’s uncovered to the minimal it must do its
task, not anything extra.
src/restaurantRatings/controller.ts…
interface Eating place { identity: string; title: string; } interface Dependencies { getTopRestaurants(town: string): Promise<Eating place[]>; } export const createTopRatedHandler = (dependencies: Dependencies) => { const { getTopRestaurants } = dependencies; go back async (request: Request, reaction: Reaction) => { const town = request.params["city"] reaction.contentType("utility/json"); const eating places = anticipate getTopRestaurants(town); reaction.standing(200).ship({ eating places }); }; };
For individuals who like to visualise these items, we will visualize the manufacturing
code as far as the handler operate that calls for one thing that
implements the getTopRatedRestaurants
interface the use of
a ball and socket notation.
The exams create this operate and a stub for the specified
operate. I will be able to display this through the use of a distinct color for the exams, and
the socket notation to turn implementation of an interface.
This controller
module is brittle at this level, so I’m going to wish to
flesh out my exams to hide choice code paths and edge instances, however that is slightly past
the scope of the item. In case you are occupied with seeing a extra thorough test and the resulting controller module, each are to be had in
the GitHub repo.
Digging into the area
At this degree, I’ve a controller that calls for a operate that does not exist. My
subsequent step is to supply a module that may satisfy the getTopRestaurants
contract. I’m going to get started that procedure through writing a large clumsy unit check and
refactor it for readability later. It is just at this level I get started considering
about how one can enforce the contract I’ve up to now established. I am going
again to my unique acceptance standards and check out to minimally design my
module.
check/restaurantRatings/topRated.spec.ts…
describe("The highest rated eating place checklist", () => { it("is calculated from our proprietary rankings set of rules", async () => { const rankings: RatingsByRestaurant[] = [ { restaurantId: "restaurant1", ratings: [ { rating: "EXCELLENT", }, ], }, { restaurantId: "restaurant2", rankings: [ { rating: "AVERAGE", }, ], }, ]; const ratingsByCity = [ { city: "vancouverbc", ratings, }, ]; const findRatingsByRestaurantStub: (town: string) => Promise< ➀ RatingsByRestaurant[] > = (town: string) => { go back Promise.unravel( ratingsByCity.clear out(r => r.town == town).flatMap(r => r.rankings), ); }; const calculateRatingForRestaurantStub: ( ➁ rankings: RatingsByRestaurant, ) => quantity = rankings => { // I do not understand how that is going to paintings, so I'm going to use a dumb however predictable stub if (rankings.restaurantId === "restaurant1") { go back 10; } else if (rankings.restaurantId == "restaurant2") { go back 5; } else { throw new Error("Unknown eating place"); } }; const dependencies = { ➂ findRatingsByRestaurant: findRatingsByRestaurantStub, calculateRatingForRestaurant: calculateRatingForRestaurantStub, }; const getTopRated: (town: string) => Promise<Eating place[]> = topRated.create(dependencies); const topRestaurants = anticipate getTopRated("vancouverbc"); be expecting(topRestaurants.period).toEqual(2); be expecting(topRestaurants[0].identity).toEqual("restaurant1"); be expecting(topRestaurants[1].identity).toEqual("restaurant2"); }); }); interface Eating place { identity: string; } interface RatingsByRestaurant { ➃ restaurantId: string; rankings: RestaurantRating[]; } interface RestaurantRating { score: Ranking; } export const score = { ➄ EXCELLENT: 2, ABOVE_AVERAGE: 1, AVERAGE: 0, BELOW_AVERAGE: -1, TERRIBLE: -2, } as const; export variety Ranking = keyof typeof score;
I’ve offered numerous new ideas into the area at this level, so I’m going to take them one by one:
- I want a “finder” that returns a suite of rankings for each and every eating place. I’m going to
get started through stubbing that out. - The acceptance standards give you the set of rules that may force the full score, however
I make a choice to forget about that for now and say that, by some means, this team of rankings
will give you the general eating place score as a numeric worth. - For this module to operate it is going to depend on two new ideas:
discovering the rankings of a cafe, and for the reason that set or rankings,
generating an general score. I create any other “dependencies” interface that
contains the 2 stubbed purposes with naive, predictable stub implementations
to stay me transferring ahead. - The
RatingsByRestaurant
represents a selection of
rankings for a specific eating place.RestaurantRating
is a
unmarried such score. I outline them inside my check to signify the
aim of my contract. Those varieties would possibly disappear sooner or later, or I
would possibly advertise them into manufacturing code. For now, it is a excellent reminder of
the place I am headed. Varieties are very reasonable in a structurally-typed language
like Typescript, so the price of doing so may be very low. - I additionally want
score
, which, in step with the ACs, consists of five
values: “superb (2), above moderate (1), moderate (0), underneath moderate (-1), horrible (-2)”.
This, too, I can seize inside the check module, ready till the “closing accountable second”
to make a decision whether or not to tug it into manufacturing code.
As soon as the fundamental construction of my check is in position, I attempt to make it collect
with a minimalist implementation.
src/restaurantRatings/topRated.ts…
interface Dependencies {} export const create = (dependencies: Dependencies) => { ➀ go back async (town: string): Promise<Eating place[]> => []; }; interface Eating place { ➁ identity: string; } export const score = { ➂ EXCELLENT: 2, ABOVE_AVERAGE: 1, AVERAGE: 0, BELOW_AVERAGE: -1, TERRIBLE: -2, } as const; export variety Ranking = keyof typeof score;
- Once more, I exploit my in part implemented operate
manufacturing unit development, passing in dependencies and returning a operate. The check
will fail, in fact, however seeing it fail in the way in which I be expecting builds my self belief
that it’s sound. - As I start enforcing the module below check, I establish some
area items that are supposed to be promoted to manufacturing code. Specifically, I
transfer the direct dependencies into the module below check. The rest that is not
a right away dependency, I depart the place it’s in check code. - I additionally make one anticipatory transfer: I extract the
Ranking
variety into
manufacturing code. I think at ease doing so as a result of this can be a common and specific area
thought. The values had been particularly referred to as out within the acceptance standards, which says to
me that couplings are much less more likely to be incidental.
Realize that the categories I outline or transfer into the manufacturing code are no longer exported
from their modules. That may be a planned selection, one I’m going to talk about in additional intensity later.
Suffice it to mention, I’ve but to make a decision whether or not I need different modules binding to
those varieties, developing extra couplings that would possibly turn out to be unwanted.
Now, I end the implementation of the getTopRated.ts
module.
src/restaurantRatings/topRated.ts…
interface Dependencies { ➀ findRatingsByRestaurant: (town: string) => Promise<RatingsByRestaurant[]>; calculateRatingForRestaurant: (rankings: RatingsByRestaurant) => quantity; } interface OverallRating { ➁ restaurantId: string; score: quantity; } interface RestaurantRating { ➂ score: Ranking; } interface RatingsByRestaurant { restaurantId: string; rankings: RestaurantRating[]; } export const create = (dependencies: Dependencies) => { ➃ const calculateRatings = ( ratingsByRestaurant: RatingsByRestaurant[], calculateRatingForRestaurant: (rankings: RatingsByRestaurant) => quantity, ): OverallRating[] => ratingsByRestaurant.map(rankings => { go back { restaurantId: rankings.restaurantId, score: calculateRatingForRestaurant(rankings), }; }); const getTopRestaurants = async (town: string): Promise<Eating place[]> => { const { findRatingsByRestaurant, calculateRatingForRestaurant } = dependencies; const ratingsByRestaurant = anticipate findRatingsByRestaurant(town); const overallRatings = calculateRatings( ratingsByRestaurant, calculateRatingForRestaurant, ); const toRestaurant = (r: OverallRating) => ({ identity: r.restaurantId, }); go back sortByOverallRating(overallRatings).map(r => { go back toRestaurant(r); }); }; const sortByOverallRating = (overallRatings: OverallRating[]) => overallRatings.type((a, b) => b.score - a.score); go back getTopRestaurants; }; //SNIP ..
Having completed so, I’ve
- stuffed out the Dependencies variety I modeled in my unit check
- offered the
OverallRating
variety to seize the area thought. This generally is a
tuple of eating place identity and a bunch, however as I mentioned previous, varieties are reasonable and I imagine
the extra readability simply justifies the minimum value. - extracted a few varieties from the check that are actually direct dependencies of my
topRated
module - finished the easy good judgment of the main operate returned through the manufacturing unit.
The dependencies between the principle manufacturing code purposes seem like
this
When together with the stubs equipped through the check, it appears ike this
With this implementation entire (for now), I’ve a passing check for my
major area operate and one for my controller. They’re totally decoupled.
Such a lot so, actually, that I think the wish to turn out to myself that they are going to
paintings in combination. It is time to get started composing the devices and development towards a
greater entire.
Starting to twine it up
At this level, I’ve a choice to make. If I am development one thing
quite straight-forward, I would possibly make a choice to dispense with a test-driven
method when integrating the modules, however on this case, I will proceed
down the TDD trail for 2 causes:
- I need to center of attention at the design of the integrations between modules, and writing a check is a
excellent instrument for doing so. - There are nonetheless a number of modules to be carried out earlier than I will be able to
use my unique acceptance check as validation. If I wait to combine
them till then, I would possibly have so much to untangle if a few of my underlying
assumptions are mistaken.
If my first acceptance check is a boulder and my unit exams are pebbles,
then this primary integration check could be a fist-sized rock: a corpulent check
exercising the decision trail from the controller into the primary layer of
area purposes, offering check doubles for the rest past that layer. No less than this is how
it is going to get started. I would possibly proceed integrating next layers of the
structure as I am going. I additionally would possibly make a decision to throw the check away if
it loses its software or is getting into my method.
After preliminary implementation, the check will validate little greater than that
I have stressed out the routes appropriately, however will quickly quilt calls into
the area layer and validate that the responses are encoded as
anticipated.
check/restaurantRatings/controller.integration.spec.ts…
describe("the controller height rated handler", () => { it("delegates to the area height rated good judgment", async () => { const returnedRestaurants = [ { id: "r1", name: "restaurant1" }, { id: "r2", name: "restaurant2" }, ]; const topRated = () => Promise.unravel(returnedRestaurants); const app = categorical(); ratingsSubdomain.init( app, productionFactories.replaceFactoriesForTest({ topRatedCreate: () => topRated, }), ); const reaction = anticipate request(app).get( "/vancouverbc/eating places/really useful", ); be expecting(reaction.standing).toEqual(200); be expecting(reaction.get("content-type")).toBeDefined(); be expecting(reaction.get("content-type").toLowerCase()).toContain("json"); const payload = reaction.frame as RatedRestaurants; be expecting(payload.eating places).toBeDefined(); be expecting(payload.eating places.period).toEqual(2); be expecting(payload.eating places[0].identity).toEqual("r1"); be expecting(payload.eating places[1].identity).toEqual("r2"); }); }); interface RatedRestaurants { eating places: { identity: string; title: string }[]; }
Those exams can get a bit of unpleasant since they depend closely on the net framework. Which
ends up in a 2d resolution I have made. I may use a framework like Jest or Sinon.js and
use module stubbing or spies that give me hooks into unreachable dependencies like
the topRated
module. I do not specifically need to reveal the ones in my API,
so the use of trying out framework trickery could be justified. However on this case, I have determined to
supply a extra typical access level: the non-compulsory selection of manufacturing unit
purposes to override in my init()
operate. This offers me with the
access level I want right through the advance procedure. As I growth, I would possibly make a decision I do not
want that hook anymore wherein case, I’m going to do away with it.
Subsequent, I write the code that assembles my modules.
src/restaurantRatings/index.ts…
export const init = ( categorical: Specific, factories: Factories = productionFactories, ) => { // TODO: Cord in a stub that fits the dependencies signature for now. // Exchange this after we construct our further dependencies. const topRatedDependencies = { findRatingsByRestaurant: () => { throw "NYI"; }, calculateRatingForRestaurant: () => { throw "NYI"; }, }; const getTopRestaurants = factories.topRatedCreate(topRatedDependencies); const handler = factories.handlerCreate({ getTopRestaurants, // TODO: <-- This line does no longer collect at this time. Why? }); categorical.get("/:town/eating places/really useful", handler); }; interface Factories { topRatedCreate: typeof topRated.create; handlerCreate: typeof createTopRatedHandler; replaceFactoriesForTest: (replacements: Partial<Factories>) => Factories; } export const productionFactories: Factories = { handlerCreate: createTopRatedHandler, topRatedCreate: topRated.create, replaceFactoriesForTest: (replacements: Partial<Factories>): Factories => { go back { ...productionFactories, ...replacements }; }, };
Every now and then I’ve a dependency for a module outlined however not anything to meet
that contract but. This is utterly fantastic. I will be able to simply outline an implementation inline that
throws an exception as within the topRatedHandlerDependencies
object above.
Acceptance exams will fail however, at this degree, this is as I’d be expecting.
Discovering and solving an issue
The cautious observer will understand that there’s a collect error on the level the
is built as a result of I’ve a warfare between two definitions:
topRatedHandler
- the illustration of the eating place as understood through
controller.ts
- the eating place as outlined in
topRated.ts
and returned
throughgetTopRestaurants
.
The reason being easy: I’ve but so as to add a title
box to the
variety in
Eating placetopRated.ts
. There’s a
trade-off right here. If I had a unmarried variety representing a cafe, moderately than one in each and every module,
I’d simplest have so as to add title
as soon as, and
each modules would collect with out further adjustments. However,
I make a choice to stay the categories separate, despite the fact that it creates
additional template code. Through keeping up two distinct varieties, one for each and every
layer of my utility, I am a lot much less more likely to couple the ones layers
unnecessarily. No, this isn’t very DRY, however I
am regularly prepared to chance some repetition to stay the module contracts as
impartial as imaginable.
src/restaurantRatings/topRated.ts…
interface Eating place { identity: string; title: string, } const toRestaurant = (r: OverallRating) => ({ identity: r.restaurantId, // TODO: I installed a dummy worth to // get started and ensure our contract is being met // then we will upload extra to the trying out title: "", });
My extraordinarily naive resolution will get the code compiling once more, permitting me to proceed on my
present paintings at the module. I’m going to in a while upload validation to my exams that make certain that the
title
box is mapped appropriately. Now with the check passing, I transfer directly to the
subsequent step, which is to supply a extra everlasting strategy to the eating place mapping.
Attaining out to the repository layer
Now, with the construction of my getTopRestaurants
operate extra or
much less in position and short of a solution to get the eating place title, I can fill out the
toRestaurant
operate to load the remainder of the Eating place
information.
Previously, earlier than adopting this extremely function-driven taste of building, I almost definitely would
have constructed a repository object interface or stub with one way intended to load the
object. Now my inclination is to construct the minimal the I want: a
Eating place
operate definition for loading the item with out making any assumptions concerning the
implementation. That may come later when I am binding to that operate.
check/restaurantRatings/topRated.spec.ts…
const restaurantsById = new Map<string, any>([
["restaurant1", { restaurantId: "restaurant1", name: "Restaurant 1" }],
["restaurant2", { restaurantId: "restaurant2", name: "Restaurant 2" }],
]);
const getRestaurantByIdStub = (identity: string) => { ➀
go back restaurantsById.get(identity);
};
//SNIP...
const dependencies = { getRestaurantById: getRestaurantByIdStub, ➁ findRatingsByRestaurant: findRatingsByRestaurantStub, calculateRatingForRestaurant: calculateRatingForRestaurantStub, }; const getTopRated = topRated.create(dependencies); const topRestaurants = anticipate getTopRated("vancouverbc"); be expecting(topRestaurants.period).toEqual(2); be expecting(topRestaurants[0].identity).toEqual("restaurant1"); be expecting(topRestaurants[0].title).toEqual("Eating place 1"); ➂ be expecting(topRestaurants[1].identity).toEqual("restaurant2"); be expecting(topRestaurants[1].title).toEqual("Eating place 2");
In my domain-level check, I’ve offered:
- a stubbed finder for the
Eating place
- an access in my dependencies for that finder
- validation that the title suits what was once loaded from the
Eating place
object.
As with earlier purposes that load information, the
getRestaurantById
returns a price wrapped in
Promise
. Even supposing I proceed to play the little sport,
pretending that I do not understand how I can enforce the
operate, I do know the Eating place
is coming from an exterior
information supply, so I can need to load it asynchronously. That makes the
mapping code extra concerned.
src/restaurantRatings/topRated.ts…
const getTopRestaurants = async (town: string): Promise<Eating place[]> => { const { findRatingsByRestaurant, calculateRatingForRestaurant, getRestaurantById, } = dependencies; const toRestaurant = async (r: OverallRating) => { ➀ const eating place = anticipate getRestaurantById(r.restaurantId); go back { identity: r.restaurantId, title: eating place.title, }; }; const ratingsByRestaurant = anticipate findRatingsByRestaurant(town); const overallRatings = calculateRatings( ratingsByRestaurant, calculateRatingForRestaurant, ); go back Promise.all( ➁ sortByOverallRating(overallRatings).map(r => { go back toRestaurant(r); }), ); };
- The complexity comes from the truth that
toRestaurant
is asynchronous - I will be able to simply treated it within the calling code with
Promise.all()
.
I don’t need each and every of those requests to dam,
or my IO-bound quite a bit will run serially, delaying all the person request, however I wish to
block till the entire lookups are entire. Fortuitously, the Promise library
supplies Promise.all
to cave in a selection of Guarantees
right into a unmarried Promise containing a set.
With this transformation, the requests to seem up the eating place pass out in parallel. That is fantastic for
a height 10 checklist because the choice of concurrent requests is small. In an utility of any scale,
I’d almost definitely restructure my carrier calls to load the title
box by way of a database
sign up for and do away with the additional name. If that choice was once no longer to be had, for instance,
I used to be querying an exterior API, I would possibly want to batch them through hand or use an async
pool as equipped through a third-party library like Tiny Async Pool
to control the concurrency.
Once more, I replace through meeting module with a dummy implementation so it
all compiles, then get started at the code that fulfills my closing
contracts.
src/restaurantRatings/index.ts…
export const init = ( categorical: Specific, factories: Factories = productionFactories, ) => { const topRatedDependencies = { findRatingsByRestaurant: () => { throw "NYI"; }, calculateRatingForRestaurant: () => { throw "NYI"; }, getRestaurantById: () => { throw "NYI"; }, }; const getTopRestaurants = factories.topRatedCreate(topRatedDependencies); const handler = factories.handlerCreate({ getTopRestaurants, }); categorical.get("/:town/eating places/really useful", handler); };
The closing mile: enforcing area layer dependencies
With my controller and major area module workflow in position, it is time to enforce the
dependencies, specifically the database get entry to layer and the weighted score
set of rules.
This ends up in the next set of high-level purposes and dependencies
For trying out, I’ve the next association of stubs
For trying out, the entire components are created through the check code, however I
have not proven that within the diagram because of muddle.
The
procedure for enforcing those modules is follows the similar development:
- enforce a check to force out the fundamental design and a
Dependencies
variety if
one is essential - construct the fundamental logical go with the flow of the module, making the check move
- enforce the module dependencies
- repeat.
I may not stroll via all the procedure once more since I have already display the method.
The code for the modules running end-to-end is to be had in the
repo. Some facets of the general implementation require further observation.
Through now, you may be expecting my rankings set of rules to be made to be had by way of but any other manufacturing unit carried out as a
in part implemented operate. This time I selected to jot down a natural operate as an alternative.
src/restaurantRatings/ratingsAlgorithm.ts…
interface RestaurantRating { score: Ranking; ratedByUser: Person; } interface Person { identity: string; isTrusted: boolean; } interface RatingsByRestaurant { restaurantId: string; rankings: RestaurantRating[]; } export const calculateRatingForRestaurant = ( rankings: RatingsByRestaurant, ): quantity => { const trustedMultiplier = (curr: RestaurantRating) => curr.ratedByUser.isTrusted ? 4 : 1; go back rankings.rankings.scale back((prev, curr) => { go back prev + score[curr.rating] * trustedMultiplier(curr); }, 0); };
I made this option to sign that this must at all times be
a easy, stateless calculation. Had I sought after to depart a very easy pathway
towards a extra complicated implementation, say one thing subsidized through information science
fashion parameterized in line with person, I’d have used the manufacturing unit development once more.
Regularly there isn’t any proper or incorrect solution. The design selection supplies a
path, so that you could talk, indicating how I look ahead to the instrument would possibly evolve.
I create extra inflexible code in spaces that I do not believe must
exchange whilst leaving extra flexibility within the spaces I’ve much less self belief
within the course.
Some other instance the place I “depart a path” is the verdict to outline
any other RestaurantRating
variety in
ratingsAlgorithm.ts
. The sort is strictly the similar as
RestaurantRating
outlined in topRated.ts
. I
may take any other trail right here:
- export
RestaurantRating
fromtopRated.ts
and reference it at once inratingsAlgorithm.ts
or - issue
RestaurantRating
out right into a not unusual module.
You’ll regularly see shared definitions in a module referred to as
varieties.ts
, despite the fact that I desire a extra contextual title like
area.ts
which supplies some hints about the type of varieties
contained therein.
On this case, I’m really not assured that those varieties are actually the
identical. They could be other projections of the similar area entity with
other fields, and I do not need to proportion them around the
module limitations risking deeper coupling. As unintuitive as this may increasingly
appear, I imagine it’s the proper selection: collapsing the entities is
very reasonable and simple at this level. In the event that they start to diverge, I almost definitely
should not merge them anyway, however pulling them aside as soon as they’re certain
may also be very tough.
If it seems like a duck
I promised to give an explanation for why I regularly make a choice to not export varieties.
I need to make a kind to be had to any other module provided that
I’m assured that doing so may not create incidental coupling, proscribing
the power of the code to adapt. Fortuitously, Typescript’s structural or “duck” typing makes it very
simple to stay modules decoupled whilst on the identical time ensuring that
contracts are intact at collect time, despite the fact that the categories aren’t shared.
So long as the categories fit in each the caller and callee, the
code will collect.
A extra inflexible language like Java or C# forces you into making some
choices previous within the procedure. As an example, when enforcing
the rankings set of rules, I’d be pressured to take a distinct method:
- I may extract the
RestaurantRating
variety to make it
to be had to each the module containing the set of rules and the only
containing the full top-rated workflow. The drawback is that different
purposes may bind to it, expanding module coupling. - However, I may create two other
RestaurantRating
varieties, then supply an adapter operate
for translating between those two an identical varieties. This may be ok,
however it might build up the quantity of template code simply to inform
the compiler what you would like it already knew. - I may cave in the set of rules into the
topRated
module utterly, however that will give it extra
duties than I would really like.
The tension of the language can imply extra pricey tradeoffs with an
method like this. In his 2004 article on dependency
injection and repair locator patterns, Martin Fowler talks about the use of a
role interface to cut back coupling
of dependencies in Java regardless of the loss of structural varieties or first
order purposes. I’d unquestionably believe this method if I had been
running in Java.
I intend to port this mission to a number of different strongly-typed languages to peer how
neatly the development applies in different contexts. Having ported it to this point to
Kotlin and Go,
there are indicators that the development applies, however no longer with out requiring some changes. I additionally imagine
that I would possibly must port it greater than as soon as to each and every language to get a greater sense
of what changes produce the most efficient effects. Extra clarification at the possible choices I made
and my sense of the effects are documented within the respective repositories.
In abstract
Through opting for to meet dependency contracts with purposes moderately than
categories, minimizing the code sharing between modules and riding the
design via exams, I will be able to create a device composed of extremely discrete,
evolvable, however nonetheless type-safe modules. If in case you have equivalent priorities in
your subsequent mission, believe adopting some facets of the method I’ve
defined. Remember, then again, that opting for a foundational method for
your mission isn’t so simple as settling on the “easiest observe” calls for
making an allowance for different components, such because the idioms of your tech stack and the
talents of your workforce. There are lots of techniques to
put a device in combination, each and every with a posh set of tradeoffs. That makes instrument structure
regularly tough and at all times attractive. I don’t have it some other method.